In JavaScript, understanding ‘this’ keyword can be challenging for developers. In this post, we’ll learn about ‘this’ keyword and its various scenarios.
Let’s get started!🚀
What is “this”?
In JavaScript, “this” is a special keyword that refers to the current execution context which means what is happening right now.
It changes its value depending on how a function is invoked. Understanding how “this” works might seem a bit tricky, but it’ll get easier for you as we’ll explore its different situations.
So, let’s explore its different scenarios.
“this” in Global Context
When we use “this” in the global scope, then “this” refers to the global object.
For example, when we use “this” in a browser environment, then this is often the window
object.
console.log(this);
Output:

Now, what is window
object?🤔
The window
object represents that browser window which contains the document. This object provides properties and methods, by using them you can interact with the browser environment.
“this” in Regular Functions
When used in regular functions, “this” refers to the global object (window
object in a browser environment).
function myFunction() {
console.log(this);
}
myFunction();
Output:

“this” in Regular Functions (strict mode)
When we use “this” in functions, in strict mode, then “this” refers to undefined
.
"use strict";
function myFunction() {
console.log(this);
}
myFunction();
Output:

“this” in Arrow Functions
Arrow functions don’t have their own “this” context. Instead, they inherit it from their parent scope (which is called “lexical scoping”).
For example:
const myfunc = () => {
console.log(this);
};
myfunc();
Output:

In the above example, myfunc
is an arrow function. Since arrow functions inherit “this” from their parent scope, so in this case, it will be the global object (in a browser environment, window
object).
“this” in Event Handlers
When we use “this” in event handlers, then “this” refers to the DOM element that triggered the event.
For example:
<button>Click me</button>
JavaScript:
const myBtn = document.querySelector("button");
myBtn.addEventListener("click", function () {
console.log(this);
});
Output:

In the above example, when you click on the button, the event handler will execute and console.log(this)
will output the element (<button>
element) to the console because an event listener is attached to the <button>
element.
Let’s take another example.
<div>This is a div.</div>
JavaScript:
const myDiv = document.querySelector("div");
myDiv.addEventListener("mouseover", function () {
console.log(this);
});
Output:
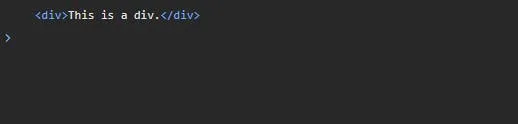
In the above example, when your cursor will be over the div
, the event handler will execute and console.log(this)
will output the element (<div>
element) to the console because an event listener is attached to the <div>
element.
“this” in Methods of an Object
When a function is a method of an object, then “this” refers to that object on which the method is called on.
Example using regular function
const myObj = {
name: "Shefali",
myMethod: function () {
console.log(this.name);
}
};
myObj.myMethod();
Output:
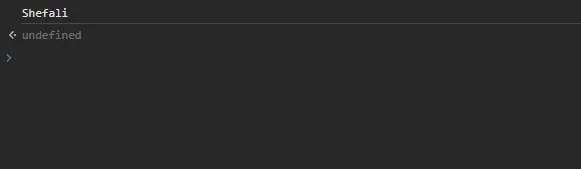
In the above example,
myObj
is an object with a propertyname
set to the string “Shefali” and with a methodmyMethod
defined using a regular function.- Inside the
myMethod
function, “this” refers to the object on which the method is called. In this case, it refers tomyObj
. - So, the output of
this.name
will beShefali
, asmyObj
has a propertyname
with the valueShefali
.
Example using arrow function
const myObj = {
name: "Shefali",
myMethod: () => {
console.log(this.name);
}
};
myObj.myMethod();
Output:

In the above example,
myObj
is an object with a propertyname
set to the string “Shefali” and with a methodmyMethod
defined using an arrow function.- Now, we are trying to log
this.name
using arrow function. - Since arrow functions inherit “this” from their parent scope, so in this case, it will be the global scope (because
myObj
is defined in the global scope). - The output of
this.name
will beundefined
, asthis.name
in the arrow function does not refer to thename
property ofmyObj
but rather to the global scope and the global scope usually does not have aname
property.
“this” in Constructors of an Object
Constructor functions are used to create objects. “this” keyword inside a constructor refers to that object which is created by that constructor.
For example:
function Person(name) {
this.name = name;
}
const myPerson = new Person("Shefali");
console.log(myPerson.name);
Output:

Let’s understand the above example,
- Here, the
Person
function is a constructor function. - In the constructor function, “this” refers to that object which is created by that constructor. In this case, when you create a new object
myPerson
usingnew Person("Shefali")
, “this” inside the constructor refers to the newly createdmyPerson
object. - When we pass the
name
parameter during object creation, this is assigned to thename
property of themyPerson
object usingthis.name = name
. - When you access
myPerson.name
, it retrieves the value of thename
property from themyPerson
object and logsShefali
to the console.
If you want to understand objects more clearly, then you can click here.
“this” in Object Prototypes
When we use “this” in object prototypes, it behaves similarly to constructors. The “this” keyword inside a prototype refers to that object which is created by that prototype.
function Person(name) {
this.name = name;
}
Person.prototype.greet = function() {
console.log(`Hello, ${this.name}!`);
};
const myPerson = new Person('Shefali');
myPerson.greet();
Output:

In the above example,
- We have a function called
Person
and we are adding a method calledgreet
to thePerson
prototype. - Using the
Person
function, we create a object calledmyPerson
with the nameShefali
. - Now we call the
greet
method on themyPerson
object. - Inside the
greet
method,this.name
refers to the name of themyPerson
object which isShefali
. - So the output will be
Hello, Shefali!
.
“this” in Class Methods
In JavaScript, the class
keyword is used to define a constructor and methods for objects. When we define a method inside a class, then “this” keyword refers to the instance of the class on which the method is called.
For example:
class Person {
constructor(name) {
this.name = name;
}
greet() {
console.log(`Hello, I'm ${this.name}!`);
}
}
const myPerson = new Person('Shefali');
myPerson.greet(); // Output: Hello, I'm Shefali!
In the above example,
- We create a new instance
myPerson
usingPerson
class. myPerson.greet()
calls thegreet
method on themyPerson
instance.- Here, “this” refers to the instance and properties of the class
Person
. - So the output is
Hello, I'm Shefali!
.
“this” in Call, Apply, or Bind Methods
JavaScript provides methods like call
, apply
, and bind
to explicitly set the value of “this” keyword in a function.
call() Method:
The call()
method is used to invoke a function with a specified value of “this” and arguments provided individually.
For example:
function greet() {
console.log(`Hello, ${this.name}!`);
}
const person = {
name: 'Shefali'
};
greet.call(person); // Output: Hello, Shefali!
In the above example,
- We have a function named
greet
and it logs a greeting message to the console using thename
property of an object. - We have an object named
person
with a propertyname
set toShefali
. - Now, the
call
method is used to invoke thegreet
function. - Here, we pass the
person
object as an argument tocall
. Which means, within thegreet
function, “this” keyword will now refer to theperson
object. - So it uses
this.name
to access thename
property of theperson
object and gives the outputHello, Shefali!
.
apply() Method:
The apply()
method is similar to call()
method. The difference is that it takes arguments as an array.
For example:
function greet(greeting, message) {
console.log(`${greeting}, ${message} ${this.name}!`);
}
const person = {
name: 'Shefali'
};
greet.apply(person, ['Hello', 'Welcome']); // Output: Hello, Welcome Shefali!
In the above example,
- We have a
greet
function, which takes two parameters,greeting
andmessage
. - We have an object named
person
with a propertyname
set toShefali
. - Now, the
apply
method is used to invoke thegreet
function. - Here, we pass the
person
object as an argument toapply
. Which means, within thegreet
function, “this” keyword will now refer to theperson
object and the array['Hello', 'Welcome']
provides values for thegreeting
andmessage
parameters. - So the function prints the
greeting
,message
, and thename
from theperson
object to the console asHello, Welcome Shefali!
.
bind() Method:
The bind()
method is used to create a new function with a specified value of “this” and initial arguments.
It doesn’t immediately invoke the function but returns a new function which we can call later.
For example:
function greet(greeting) {
console.log(`${greeting}, ${this.name}!`);
}
const person = {
name: 'Shefali'
};
const greetPerson = greet.bind(person, 'Good morning');
greetPerson(); // Output: Good morning, Shefali!
In the above example,
- We have a
greet
function, which takes a parameter,greeting
. - We have an object named
person
with a propertyname
set toShefali
. - The
bind
method is used to create a new functiongreetPerson
by associating the “this” value with theperson
object and providing a default value for thegreeting
parameter asGood morning
. - When we call
greetPerson()
, it uses thegreet
function, with value of “this” set to theperson
object and gives the output asGood morning, Shefali!
.
That’s all for today.
I hope it was helpful.
Thanks for reading.
For more content like this, click here.
You can also follow me on X(Twitter) for getting daily tips on web development.
Check out toast.log, a browser extension that lets you see errors, warnings, and logs as they happen on your site — without having to open the browser’s console. Click here to get a 25% discount on toast.log.
Keep Coding!!