In JavaScript, the console
object is a built-in feature that provides a set of methods for displaying debug information. These methods are part of every web browser and therefore easily accessible to developers. They are part of the browser’s developer tools, which can be opened using F12 or Ctrl+Shift+I (Cmd+Opt+I on Mac) in most browsers.
The console
methods are very important for debugging, logging, and giving feedback during the development process. You can output messages, objects, and other information directly to the browser console, which helps in tracking the behavior of your web application. In this blog, I’ll share the 14 most commonly used console
methods and their syntax.
Let’s get started!🚀
console.log()
This method is used to log a message to the console.
For example:
console.log("Hello, World!");
Output:

console.error()
This method is used to display error messages to the console.
It makes the message stand out by displaying it in red color (in most browsers) and helps in identifying and tracking errors easily.
For example:
console.error("This is an error message!");
Output:
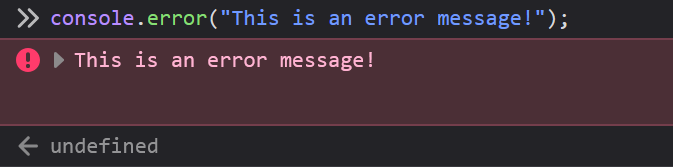
console.warn()
This method is used to display warning messages in the console.
This will display the warning message in yellow color (in most browsers), making it easy to differentiate it from regular logs.
It is often used to display potential issues that might not necessarily be errors but could lead to problems.
For example:
console.warn("This is a warning message!");
Output:
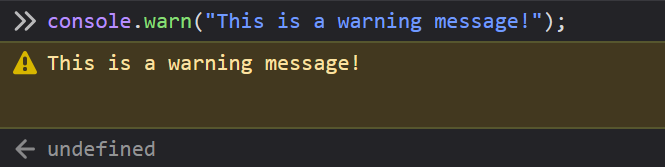
console.info()
This method is used to display informational messages in the console.
It is typically used for logging general information that may not be errors or warnings, but provide useful insights into the code flow.
For example:
console.info("This is an informational message!");
Output:
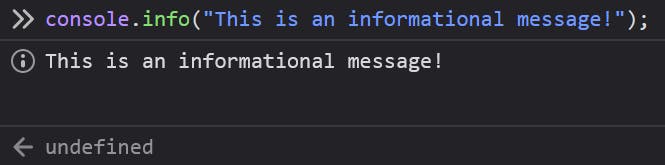
console.debug()
This method is used to display debug messages to the console.
It’s helpful for providing detailed information while debugging your code.
Syntax:
console.debug("Debugging information!");
Output:

Example:
function calculateSum(a, b) { console.debug("Function called with arguments:", a, b); return a + b; } console.log(calculateSum(5, 3)); // Logs the debug message first, then the sum.
Output:
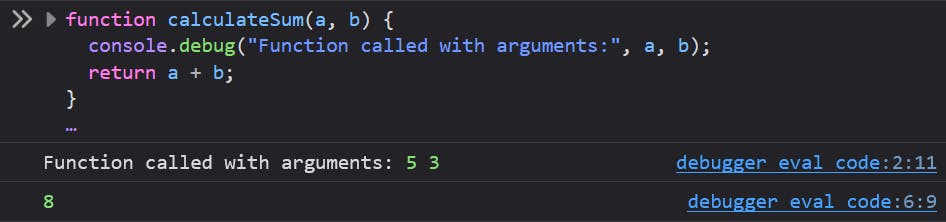
Note: Some browsers may hide console.debug()
messages in the console unless the debug level is enabled.
console.table()
You can use this method to display data in a tabular format in the console, making it easier to view and analyze data.
For example:
const users = [ { name: 'John Doe', age: 30, occupation: 'Software Developer' }, { name: 'Jane Smith', age: 25, occupation: 'Graphic Designer' }, { name: 'Mike Johnson', age: 35, occupation: 'Project Manager' }, ]; console.table(users);
Output:
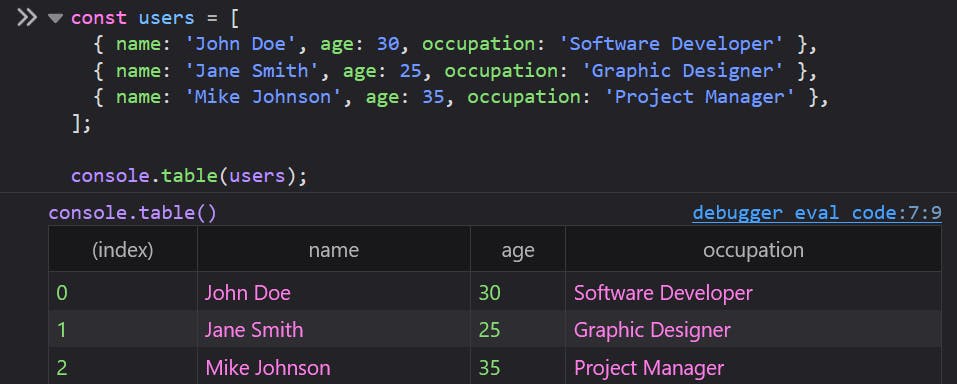
console.group()
You can use this method to create a group of related messages in the console.
This helps organize and structure logs for better readability.
For example:
console.group('User Details'); console.log('Name: John Doe'); console.log('Age: 30'); console.log('Occupation: Software Developer'); console.groupEnd();
Output:
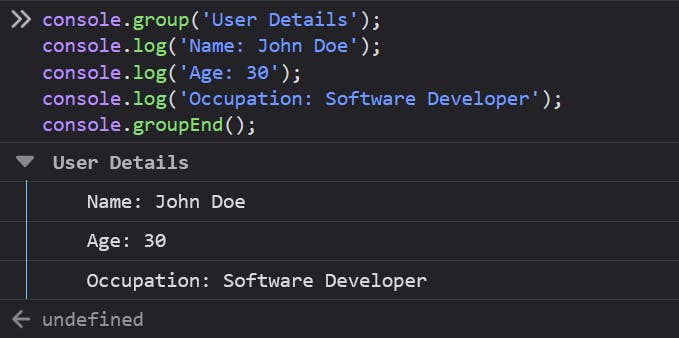
console.groupEnd()
This method is used to end a group of messages in the console that was started with console.group()
or console.groupCollapsed()
.
Example with console.group()
:
console.group('User Details'); console.log('Name: John Doe'); console.log('Age: 30'); console.log('Occupation: Software Developer'); console.groupEnd();
Output:
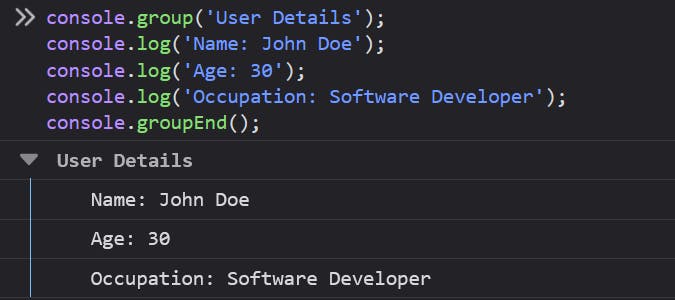
This helps in group related messages together, making them easier to read and understand.
Example with console.groupCollapsed()
:
You can also use console.groupCollapsed()
to start a collapsed group, which is hidden by default.
console.groupCollapsed('User Details'); console.log('Name: John Doe'); console.log('Age: 30'); console.log('Occupation: Software Developer'); console.groupEnd();
This will initially show the group as collapsed, allowing you to expand it when needed.
Output:
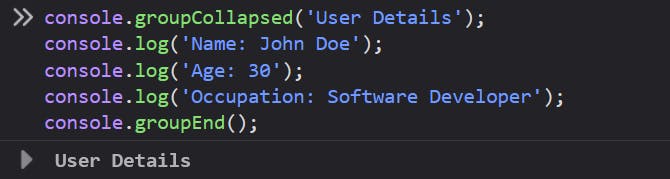
console.time() and console.timeEnd()
console.time()
and console.timeEnd()
methods are used to measure the time taken by a block of code to execute.
These methods allow you to track how long a specific operation or function takes, which is useful for debugging performance issues or optimizing your code.
For example:
console.time("timer1"); // Start the timer // Example code block to measure time for (let i = 0; i < 1000000; i++) { Math.sqrt(i); } console.timeEnd("timer1"); // End the timer and log the time
Output:
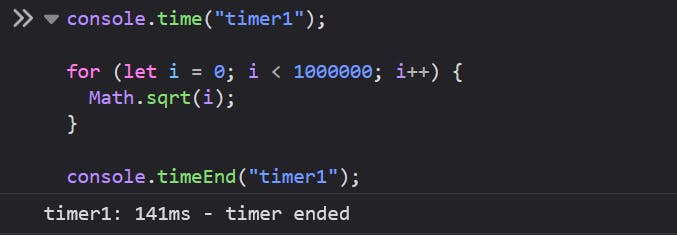
In this example, the console.time("timer1")
starts a timer, and after the loop executes, console.timeEnd("timer1")
ends the timer and prints the time taken in milliseconds.
It’s helpful for:
- When you want to measure the time taken by specific parts of your code, like loops, functions, or requests, to identify bottlenecks.
- When you want to compare the performance of different functions or algorithms.
You can have multiple timers running at the same time by using different labels.
For example:
console.time("timer1"); for (let i = 0; i < 500000; i++) { Math.sqrt(i); } console.timeEnd("timer1"); console.time("timer2"); for (let i = 0; i < 1000000; i++) { Math.sqrt(i); } console.timeEnd("timer2");
Output:
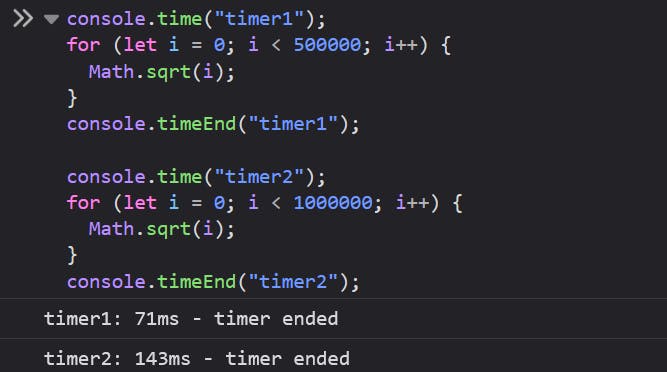
console.assert()
This method is used to test if a condition is true. If the condition is false, it logs an error message to the console. If the condition is true, nothing happens.
Syntax:
console.assert(condition, message);
condition
: The condition you want to test.message
: The message that will be displayed if the condition is false.
For example:
let age = 18; console.assert(age >= 21, "Age must be 21 or older"); // Will display an error message because the condition is false age = 22; console.assert(age >= 21, "Age must be 21 or older"); // No output because the condition is true
Output:

Since console.assert()
does not throw an error, it doesn’t interrupt your program but simply logs information if necessary.
console.count()
This method logs the number of times it has been called with the same label.
To put it simply, every time you call console.count()
with the same label, it increments the count associated with that label and logs it to the console. This is useful for tracking how many times a specific action, such as clicking a button or submitting a form, occurs in your application.
For example:
console.count("Count A"); console.count("Count A");
Output:
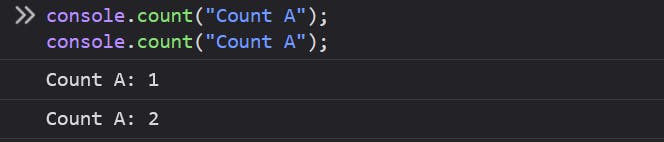
console.countReset()
This method resets the count for a specific label back to zero.
This is helpful if you want to start counting from the beginning, such as when the user navigates away from a page and then returns.
For example:
console.count("Count A"); console.countReset("Count A");
Output:
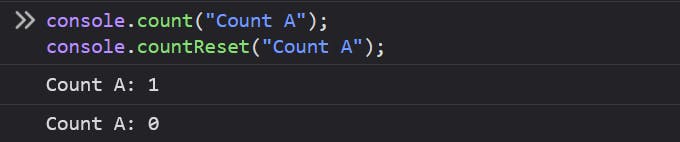
console.dir()
This method displays an interactive list of the properties of the specified JavaScript object.
This is particularly useful for inspecting objects.
For example:
const person = { name: "John", age: 30, address: { city: "New York", zip: "10001" }, greet() { console.log("Hello!"); } }; console.dir(person);
The output will display a collapsible tree of the person object with its properties and methods.
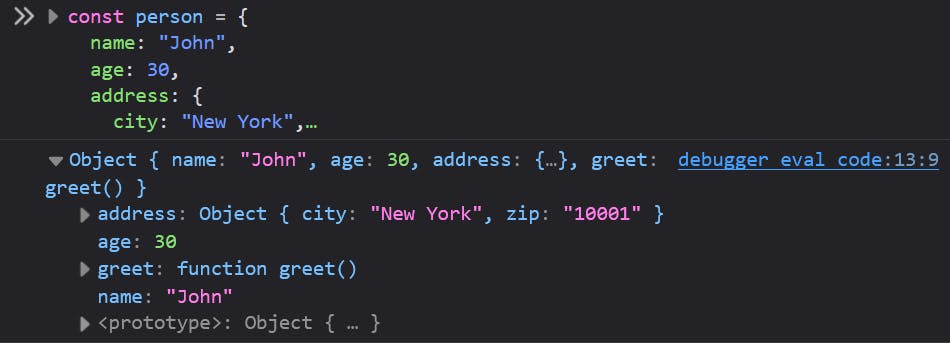
When you need to inspect the properties of an object or array in-depth, console.dir()
is more useful than console.log()
, especially for objects with deep nesting.
console.clear()
This method is used to clear the console.
By mastering these console methods, you can enhance the JavaScript development workflow and make debugging as well as managing complex applications much easier.
That’s all for today.
I hope it was helpful.
Thanks for reading.
Here are 45 More JavaScript Tips & Tricks for Developers.
For more content like this, click here.
Follow me on X(Twitter) for daily web development tips.
Keep Coding!!
Check out toast.log, a browser extension that lets you see errors, warnings, and logs as they happen on your site — without having to open the browser’s console. Click here to get a 25% discount on toast.log.